Generic Code: What It Is and What It Is Not? – Polish Dreamin’ 25
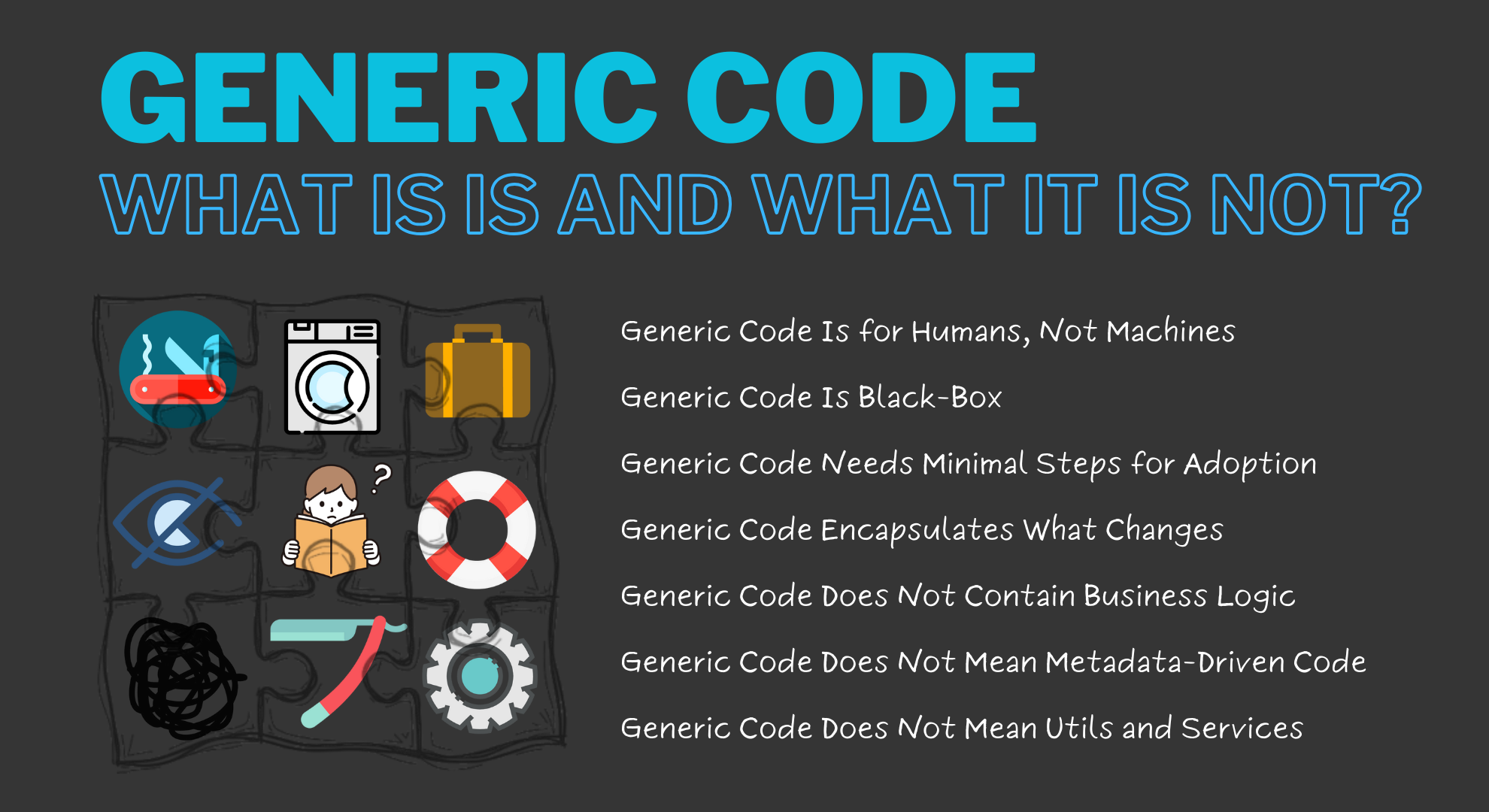
It’s not easy to answer that question directly. Instead of simply focusing on what generic code is, let’s consider another question: What traits does generic code truly have, and which are merely false impressions that make us think it’s generic?
That’s quite a mouthful, but in this post, I’ll share my personal experiences to clarify what generic code is – and what it isn’t.
Every discussion or post should have a clear goal. We can certainly talk about generic code, but why? Before diving into the development of generic code, let’s begin by understanding why we need it in the first place.
Why We Need Generic Code?
Software development is fundamentally about meeting business requirements. You receive a task from your project manager with specific acceptance criteria, turn on some background music, and start coding – let’s call this the “just resolve the problem” approach.
In this approach, you focus just on delivery.
Duplicated code? Inconsistency? It’s not your problem. Just deliver.
As an example, let’s say you might need a custom data table for Accounts, so you create an accountDatatable
LWC. Then you need one for Contacts, so you copy the code and create a contactDatatable
LWC. For Opportunities, you do the same again.
You even forgot that lightning-datatable
exists, and ended up with something like this:
<!-- accountDatatable --> <template> <lightning-card title="Accounts"> <table border="1" cellpadding="5" cellspacing="0"> <thead> <tr> <th>Name</th> <th>Industry</th> </tr> </thead> <tbody> <template for:each={accounts} for:item="acc"> <tr key={acc.Id}> <td>{acc.Name}</td> <td>{acc.Industry}</td> </tr> </template> </tbody> </table> </lightning-card> </template>
<!-- contactDatatable --> <template> <lightning-card title="Contacts"> <table border="1" cellpadding="5" cellspacing="0"> <thead> <tr> <th>Name</th> <th>Email</th> </tr> </thead> <tbody> <template for:each={contacts} for:item="con"> <tr key={con.Id}> <td>{con.Name}</td> <td>{con.Email}</td> </tr> </template> </tbody> </table> </lightning-card> </template>
Although straightforward, it can lead to several major issues:
- Duplicated code: You end up copying and pasting the same logic repeatedly.
- Inconsistency: If you update one data table, you must remember to update all the others; if you forget, the UI becomes inconsistent.
- Reinventing the wheel: Every developer in the project ends up creating a new version of the same component, wasting time and effort.
Clearly, “just resolving the problem” isn’t enough. We need a different approach.
The next approach will be called The “Abstract Problem” Approach.
Rather than focusing on the immediate task (for example, “display a list of Accounts”), think in terms of the underlying problem: “display a list of records”. Your abstraction is “records,” and your concrete use cases are Accounts, Contacts, Opportunities, and any other record type.
By identifying the abstract problem, you can devise a more robust and flexible solution. Ask yourself:
- Who will use it?
- What varies, and what stays the same?
- Which parts need to be configurable?
- What are the default settings?
- How would I like to see it used in my code?
- …and so on.
After laying this foundation, we can finally answer the question: “Why do we need generic code?”
- Repetitive Problems: Generic code tackles recurring patterns, preventing countless rewrites.
- Boosts Developer Productivity: Developers can quickly adopt a ready-made solution for multiple scenarios.
- Consistency: A single, well-designed component ensures uniform UI and logic across the application.
- Less Duplication: You maintain one codebase for common functionality instead of scattering copies everywhere.
Generic code reduces technical debt and fosters collaboration by providing a clear, reusable solution the entire team can rely on. However, it’s crucial to avoid over-engineering – don’t create abstractions for hypothetical needs. Focus on real, repeating issues to strike the right balance between flexibility and simplicity.
Ultimately, software development is about solving business problems, and generic code offers a strategic way to solve them once and apply that solution multiple times.
Now, the next big questions are:
- How do we write truly generic code?
- What does “generic” code even mean?
What Does “Generic” Code Even Mean?
Before we start. 🚨
The rules described below may seem similar, but they fit together like pieces of a puzzle – each one complements the others. You might see some of these principles repeated in slightly different contexts.
Everything you’ll read here is based on my personal experience and opinions. Don’t treat it as absolute – challenge both yourself and me. If you disagree with anything, that’s great! Feel free to message me on LinkedIn or leave a comment below.
Generic Code Is for Humans, Not Machines
aka Naming Convention
aka Encapsulation
High-level programming languages were invented to make developers’ lives easier. Low-level programming languages are extremely fast and powerful, but with greater possibilities comes greater complexity. We’ve created new programming languages to be more developer-friendly -making code easier to read and understand – at the cost of efficiency, which nowadays isn’t as critical because our computers’ processing power continues to grow according to Moore’s Law.
A computer can understand any kind of code as long as it compiles. You can write really ugly, messy code, and it will still work. The computer will simply interpret it as a stream of 0s and 1s.
When you write "generic" code, you’re not doing it for the computer – it doesn’t have feelings. You’re doing it for your future self and for other developers who might feel frustrated and annoyed because of how your "generic" code was written.
Make your code intuitive and require as little extra information as possible.
Why should you care about other developers?
It’s about cost savings: intuitive code leads to faster development, simpler maintenance, and less time spent – resulting in lower project expenses overall.
Below are some practical tips on how to achieve this.
Define the Abstraction and "Interface"
Begin by clarifying the abstract problem you want to solve. Once you’ve identified that problem, define how your code will be used – specifically, which classes, methods, and properties will be accessible to other developers. This “interface” should be intuitive and straightforward, ideally reading like plain English to convey a clear “story” of what your code does.
✅
public interface Cacheable { Boolean contains(String key); Set<String> getKeys(); Object get(String key); void put(String key, Object value); void remove(String key); }
CacheManager.ApexTransaction.contains('Key'); CacheManager.ApexTransaction.put('Key', 'My Value'); CacheManager.ApexTransaction.get('Key'); CacheManager.ApexTransaction.getKeys(); CacheManager.ApexTransaction.remove('Key');
✅
@IsTest static void calloutTest() { new HttpMock() .get('/api/v1/authorize') .body('{ "token": "aZ3Xb7Qk" }') .statusCodeOk() .post('/api/v1/create') .body('{ "success": true }') .statusCodeOk() .mock(); }
or maybe even
@IsTest static void calloutTest() { new HttpMock() .whenGetOn('/api/v1/authorize') .thenReturnBody('{ "token": "aZ3Xb7Qk" }') .withStatusCodeOk() .whenPostOn('/api/v1/create') .thenReturnBody('{ "success": true }') .withStatusCodeOk() .mock(); }
✅
SOQL.of(Account.SObjectType) .byRecordType('Partner') .toValueOf(Account.Name);
Ask for Less
Require Only What’s Necessary
Make it easy for your fellow developers to use your code. Don’t ask for things you can determine yourself; only request what’s truly necessary.
❌
import { LightningElement, api } from 'lwc'; export default class RecordBanner extends LightningElement { @api record; // ... }
In this example, it’s unclear what the record
object should contain or which fields and structure are required. Developers must read the implementation to figure it out, which can be time-consuming.
✅
import { LightningElement, api } from 'lwc'; export default class RecordBanner extends LightningElement { @api recordId; // ... }
Here, you don’t need to worry about the internal implementation; you just provide a recordId
. The component can automatically determine the SObjectType
and fetch the relevant fields, while still offering the option for further customization if needed. The initial setup remains extremely simple.
Replace Method Parameters with Clear Method Name
Instead of asking for a parameter, use descriptive method names. The name itself can indicate the value of the parameter.
public without sharing virtual class RecordBuilder { public SObject build() { // ... } // ❌ public SObject build(Boolean isInsert) { // ... } // ✅ public SObject buildAndInsert() { return this.build(true); } }
public class AccountSelector { // ❌ public List<Account> getByRecordType(String recordType) { // ... } // ✅ public List<Account> getParentAccounts() { return this.getByRecordType('Partner_Account_RT_1'); } }
public class IntegrationService { // ❌ public String getAccessToken(String namedCredentials, String path) { // ... } // ✅ public String getMulesoftAccessToken() { return this.getByRecordType('Mulesoft_Named_Credential', '/v1/token'); } public String getAwsAccessToken() { return this.getByRecordType('AWS_NC_1', '/v1/auth'); } }
By naming methods in a more descriptive way, you streamline code usage and reduce the need for additional parameters. This approach promotes clarity and maintainability across your project.
Generic Code Does Not Require a Detailed Understanding of Its Internal Implementation
aka Encapsulation
aka Dependency Inversion Principle
aka Black-Box
Let’s begin with Cognitive Complexity, a term originating in psychology that describes how individuals perceive and interpret the world around them.
In software development, Cognitive Complexity measures how difficult it is to intuitively understand a piece of code (such as a function or class).
Less Cognitive Complexity → More Readability
Low cognitive complexity is especially important when writing generic code. Developers should strive to make their code as easy to understand as possible. Do you need to fully understand generic code? Of course no. Protect your cognitive capacity.
Generic code should function like a black box: you shouldn’t need to dive into its internal implementation to grasp how it works. Instead, it should present a clear "interface/API" that you can interact with.
Consider how we use a computer. Most of us have a high-level idea of how it operates, but we don’t know every detail. The computer provides interfaces – like a keyboard, mouse, and display – that let us work with it. That’s exactly how your generic code should behave.
How do you create this Black Box?
Use Encapsulation
Encapsulation is the ability of an object to hide parts of its state and behaviors from other objects, exposing only a limited interface to the rest of the program.
How to provide encapsulate your code?
Encapsulation is often provided through interfaces in most programming languages. For example:
public interface Cacheable { Boolean contains(String key); Set<String> getKeys(); Object get(String key); void put(String key, Object value); void remove(String key); }
A common mistake when using encapsulation is defining an interface but continuing to call the concrete implementation directly, rather than relying on the interface itself. This practice violates the Dependency Inversion Principle (DIP), which states that classes should depend on high-level abstractions (interfaces) rather than concrete implementations.
public interface Queryable { // ... } public virtual inherited sharing class SOQL implements Queryable { // ... }
❌ Incorrect Usage
SOQL query = SOQL.of(Account.SObjectType) .with(Account.Id, Account.Name, Account.Industry);
In this example, the code directly references the SOQL class instead of working through the Queryable interface.
✅ Correct Usage
Queryable query = SOQL.of(Account.SObjectType) .with(Account.Id, Account.Name, Account.Industry);
Here, we depend on the interface (Queryable
), maintaining proper encapsulation and adhering to Dependency Inversion Principle.
Work with an interface
When implementing the builder pattern, always return the interface rather than the concrete class. This ensures callers can only access the interface’s methods, helping maintain loose coupling, encapsulation, and adherence to best practices like the Dependency Inversion Principle.
Remember: If you return the concrete class, consumers of your builder will have access to every public member, not just those defined in the interface.
public interface RecordBuilder { RecordBuilder set(SObjectField field, Object value); RecordBuilder setRelationship(String relationship, Object value); // ... }
❌ Incorrect Usage
public class AccountBuilder implements RecordBuilder { // ... public AccountBuilder set(SObjectField field, Object value) { // ... return this; } public AccountBuilder setRelationship(String relationship, Object value) { // ... return this; } }
In this example, returning AccountBuilder
allows developers to access any public methods or properties of the AccountBuilder class, bypassing the abstraction provided by the RecordBuilder
interface.
✅ Correct Usage
public class AccountBuilder implements RecordBuilder { // ... public RecordBuilder set(SObjectField field, Object value) { // ... return this; } public RecordBuilder setRelationship(String relationship, Object value) { // ... return this; } }
By returning RecordBuilder
, you ensure callers are restricted to the interface’s methods – even if your concrete class contains additional public methods. This promotes a clean, consistent API and makes future maintenance easier.
Use Access Modifiers
Hide what’s needed by using private
. Expose what can be called using public
(Apex) or @api
(LWC).
import { LightningElement, api } from 'lwc'; export default class CustomIcon extends LightningElement { @api url; @api color = '#fff'; @api size = 'medium'; @api title = ''; @api alternativeText = ''; _background; _state; }
public with sharing class ProfileInfo { // properties public static Profile getByName(String profileName) { // ... return profile; } private static Profile cacheProfile(String profileName) { // ... } }
Make your code easy to use by clearly indicating which methods and properties are publicly available, and hide the rest.
Define the Interface at the Start of the Class
One effective practice is to declare your interface at the top of the class. This approach immediately highlights which methods are publicly available, sparing developers from having to sift through the entire implementation to find them. It also clarifies the "contract" that any concrete class must fulfill, promoting consistency and easier maintenance.
public virtual inherited sharing class SOQL implements Queryable { public interface Queryable { // SELECT // ... Queryable with(List<SObjectField> fields); Queryable with(Iterable<String> fields); Queryable with(String fields); // ... // USING SCOPE Queryable delegatedScope(); Queryable mineScope(); Queryable mineAndMyGroupsScope(); Queryable myTerritoryScope(); Queryable myTeamTerritoryScope(); Queryable teamScope(); // WHERE Queryable whereAre(FilterGroup filterGroup); Queryable whereAre(Filter filter); Queryable whereAre(String conditions); Queryable conditionLogic(String order); Queryable anyConditionMatching(); // ... } }
Generic Code Needs Minimal Steps for Adoption
aka Keep It Simple, Stupid (KISS)
aka Hick’s Law
I love board games. There are many different types: some have 50 pages of instructions, while others are relatively easy to understand. However, the best board games follow the principle: “Easy to learn, hard to master.” That means the game’s rules are simple – after a few minutes, you can start playing, but to improve, you need to build your own strategy based on those simple rules.
In programming, we can say this:
“The best code is easy to start and hard easy to configure later.”
What does that mean?
I hate it when I pick up a library and need 10,000 configurations just to use it. After a few minutes, I’m like, “Nah, it’s not for me.” Maybe I’m lazy, but remember that laziness often leads to productivity improvements.
Write code so its basic usage is very simple. How do we do that?
Single Class
If you’ve created a library, try keeping it in a single class. As Robert Sösemann once said: “Single-class libraries are sexy,” and I couldn’t agree more. Some might contend that reading thousands of lines in one Apex class is cumbersome. In truth, you can still break your code into smaller subclasses while maintaining a single primary class as the entry point. The goal is to ensure that nobody has to dive into the internal workings to understand how everything fits together.
A single class can often be copied and pasted, making it incredibly straightforward to start using your code. It also offers a single point of entry for developers. If you’re still not convinced, consider placing related files in a separate directory to keep your codebase organized.
Separate Directory
If a single class still doesn’t sound ideal, don’t worry. You can place your code in a separate folder in your repository – such as platform/main/classes/my-lib
– or at least add a prefix to your classes so they’re easy to locate and manage.
Working in a large, monolithic repository can be challenging. Avoid placing business logic in the platform
folder. It should contain only the logic that the entire team can use.
Why Is It Important?
To use generic code, people need to be able to find it. Of course, you could create a list of all libraries and generic solutions in Confluence, but remember – it should be convenient for others. Searching for a link and navigating through Confluence adds extra steps. By placing all generic code in a platform folder, it becomes much easier to locate – and therefore use.
Default Configurations
Provide default configurations for almost everything. This approach lets developers get started quickly, while still allowing them to override defaults later as needed.
One of my favorite examples is seen in LWC components.
❌
import { LightningElement, api } from 'lwc'; export default class GenericDataTable extends LightningElement { @api title; @api variant; @api columns; @api actions; @api data; @api keyField; }
What’s required to start using this component?
You have to pass multiple parameters – perhaps you don’t even know the data format yet, so you’ll spend time figuring it out. This can be frustrating.
❌
<template> <c-generic-data-table title="Component Titile" variant="basic" columns={columns} data={data} actions={actions} key-field="id" > </c-generic-data-table> </template>
✅
import { LightningElement, api } from 'lwc'; const VARIANTS = { BASIC: 'BASIC', BRAND: 'BRAND', OUTLINE: 'OUTLINE' }; const ACTIONS = { VIEW: 'VIEW', REMOVE: 'REMOVE' }; export default class GenericDataTable extends LightningElement { @api columns = []; @api data = []; @api title = 'Component Title'; @api variant = VARIANTS.BASIC; @api actions = [ACTIONS.VIEW]; @api keyField = 'id'; }
What’s required to start using this component now?
Almost nothing. Just add the component to your LWC, specify the columns and data (only what’s truly required), and you’ll immediately see results. Any additional configuration can be added step by step.
<template> <c-generic-data-table columns={columns} data={data} > </c-generic-data-table> </template>
<template> <c-generic-data-table title="My Title" columns={columns} data={data} > </c-generic-data-table> </template>
This approach is directly connected to the Ask For Less principle.
Generic Code Encapsulates What Changes
aka Encapsulation (once again)
aka Open-Closed Principle
aka Design Patterns
Pattern, pattern, patterns everywhere.
Let’s start with a short story about shipping containers:
Before containerization, cargo was handled manually as break bulk, requiring multiple loadings and unloadings at warehouses and ports. This process was costly, slow, and prone to damage. Malcolm McLean’s invention of the standardized shipping container drastically reduced these inefficiencies, enabling goods to move seamlessly between ships, trucks, and trains. Today, more than 17 million containers facilitate around 200 million trips each year, revolutionizing global trade with lower costs, faster transit, and enhanced security.
What did Malcolm McLean do?
He identified a problem (chaotic shipping), discovered a pattern, and created a standardized solution.
The best code I’ve seen identifies patterns effectively – distinguishing what stays the same and what changes.
We’ve already discussed encapsulation, but let’s examine it from a different perspective. In this section, we’ll focus on “generic” code that can be extended. To design such code, we must identify what varies and handle everything else.
Example 1 – Data Factory
Step 0 – One class, separated method per object.
// DataFactory.cls public class DataFactory { public static Account createAccount() { Account account = new Account( Name = 'My Account', Email = 'myAccount@email.com' ); insert account; return account; } public static Contact createContact() { Contact contact = new Contact( LastName = 'My Contact' ); insert contact; return contact; } }
This approach works – it’s simple and straightforward. However, what happens when more and more methods for other objects are added? You risk overloading the class, causing merge conflicts, making the code difficult to read, and creating a “god class.”
Additionally, if you need an Account or Contact without inserting it into the database, do you create a separate method or introduce a parameter like Boolean doInsert
? Even though this code is simple, it can lead to maintainability issues down the road.
Step 1 – Introduce an Interface and Create Separate Factories per Object
// DataFactory.cls public interface DataFactory { sObject getRecord(); sObject createRecord(); }
// AccountFactory.cls public class AccountFactory implements DataFactory { public sObject getRecord() { return new Account( Name = 'My Account' ); } public sObject createRecord() { Account account = getRecord(); insert account; return acccount; } }
// ContactFactory.cls public class ContactFactory implements DataFactory { public sObject getRecord() { return new Contact( LastName = 'My Contact' ); } public sObject createRecord() { Contact contact = getRecord(); insert contact; return contact; } }
// DataCreator.cls public class DataCreator { private final Map<sObjectType, System.Type> OBJECT_TO_FACTORY = new Map<sObjectType, System.Type>{ Account.sObjectType => AccountFactory.class, Contact.sObjectType => ContactFactory.class }; public static Object createRecord(sObjectType objectTypeToCreate) { DataFactory factory = (DataFactory) OBJECT_TO_FACTORY.get(objectTypeToCreate).newInstance(); return factory.createRecord(); } }
This approach follows the Single Responsibility Principle: each class is in charge of data creation for one object type, and all share the same interface. This makes them easy to use from the DataCreator class, reducing merge conflicts and streamlining development.
However, we can still improve it. For instance, what if you need an account or contact without inserting it into the database? Do you need a separate method? Should you add a parameter like Boolean doInsert
? Although this code works, it may lead to future challenges.
Step 3 – Abstract Class with Shared Logic
public abstract class DataFactory { public abstract sObject getRecord(); // <== this varies public sObject createRecord() { // <== this is the same sObject record = this.getRecord(); insert record; return record; }; }
public class AccountFactory extends DataFactory { public override sObject getRecord() { return new Account( Name = 'My Account' ); } }
public class ContactFactory extends DataFactory { public override sObject getRecord() { return new Contact( LastName = 'My Contact' ); } }
public class DataCreator { private final Map<sObjectType, System.Type> OBJECT_TO_FACTORY = new Map<sObjectType, System.Type>{ Account.sObjectType => AccountFactory.class, Contact.sObjectType => ContactFactory.class }; public static Object createRecord(sObjectType objectTypeToCreate) { DataFactory factory = (DataFactory) OBJECT_TO_FACTORY.get(objectTypeToCreate).newInstance(); return factory.createRecord(); } }
Each specific implementation now only defines what changes (getRecord()
), while insertion is handled universally in the abstract class.
But what if you want to create a record without inserting, or override certain fields?
Step 4 – Expanding the Functionality
public abstract class DataFactory implements Factory { public interface Factory { Factory withFieldValue(SObjectField field, Object value); Factory withFieldValue(Map<SObjectField field, Object value> valuePerField); Object get(); Object get(Integer recordsAmount); Object put(); // with insert Object put(Integer recordsAmount); // with insert } public abstract sObject getRecord(); // <== this varies // this is the same public Factory withFieldValue(SObjectField field, Object value) { // ... return this; } // this is the same public Factory withFieldValue(Map<SObjectField field, Object value> valuePerField) { // ... return this; } public Object get() { // <== this is the same // ... } public Object get(Integer recordsAmount) { // <== this is the same // ... } public Object put() { // <== this is the same // ... } public Object put(Integer recordsAmount) { // <== this is the same // ... } }
public class AccountFactory extends DataFactory { public override sObject getRecord() { return new Account( Name = 'My Account' ); } }
public class ContactFactory extends DataFactory { public override sObject getRecord() { return new Contact( LastName = 'My Contact' ); } }
public class DataCreator { private final Map<sObjectType, System.Type> OBJECT_TO_FACTORY = new Map<sObjectType, System.Type>{ Account.sObjectType => AccountFactory.class, Contact.sObjectType => ContactFactory.class }; public static DataFactory.Factory get(sObjectType objectTypeToCreate) { return (DataFactory.Factory) OBJECT_TO_FACTORY.get(objectTypeToCreate).newInstance(); } }
Usage
DataCreator.get(Account.SObjectType).get(); DataCreator.get(Account.SObjectType).put(10); DataCreator.get(Account.SObjectType) .withFieldValue(Account.Name, 'Test') .withFieldValue(Account.Source, 'Blog') .put();
In this final approach, we’ve identified the pattern of record creation and isolated what varies (object type, default field values). We also provide options for inserting the records or just returning them. Need an OpportunityFactory
? Simply create a class that extends DataFactory
and register it in DataCreator
.
Example 2 – Selectors
The same principle applies to selectors. What’s the difference between various selectors? Primarily, the SObjectType
. Of course, you can configure other variable elements like field-level security, sharing settings, or default fields. However, if we follow the minimal steps principle, only the SObjectType is needed to get the code working right away.
public inherited sharing class SOQL_Account extends SOQL implements SOQL.Selector { public static SOQL_Account query() { return new SOQL_Account(); } private SOQL_Account() { super(Account.SObjectType); // <== only SObjectType varies } }
public inherited sharing class SOQL_Contact extends SOQL implements SOQL.Selector { public static SOQL_Contact query() { return new SOQL_Contact(); } private SOQL_Contact() { super(Contact.SObjectType); // <== only SObjectType varies } }
Generic code only asks you to specify what varies; it handles everything else – common across all use cases – inside the generic structure.
How to encapsulate what changes?
Object-Oriented Programming
Place shared functionality in an abstract or virtual base class, and define abstract or virtual methods (or interfaces) for the parts that differ.
public abstract class DataFactory { public abstract sObject getRecord(); public sObject createRecord() { sObject record = this.getRecord(); insert record; return record; }; }
Learn more about Abstract, Virtual, Interface in Apex.
Dependency Injection (DI)
Dependency Injection (DI) is a programming technique that lets objects receive their dependencies from outside, rather than creating them internally. This separates the construction of objects from their usage, making code more modular and easier to maintain.
public interface PaymentGateway { Decimal processPayment(Decimal amount); }
public with sharing class PaymentService { private PaymentGateway gateway; public PaymentService(PaymentGateway gateway) { this.gateway = gateway; } public Decimal makePayment(Decimal amount) { return gateway.processPayment(amount); } }
In this setup, PaymentService
contains the shared logic (e.g., paying invoices), while the Gateway itself is provided (injected) from the outside.
What varies – different payment methods – goes into separate classes:
public with sharing class PayPalGateway implements PaymentGateway { public Decimal processPayment(Decimal amount) { return amount * 0.97; } } public with sharing class StripeGateway implements PaymentGateway { public Decimal processPayment(Decimal amount) { return amount * 0.98; } }
Need another payment gateway? You don’t touch PaymentService
at all – just create a new class:
public with sharing class AppleGateway implements PaymentGateway { public Decimal processPayment(Decimal amount) { return amount * 0.97; } }
Because the PaymentService
only depends on the PaymentGateway
interface, it’s easy to add or swap implementations without modifying the existing service logic.
Design Patterns
Many design patterns address specific problems by isolating variability, making your code more flexible and maintainable.
Generic Code Does Not Contain Business Logic
aka Open-Closed Principle
Generic code should avoid containing business logic – at least not directly. Its main purpose is to solve abstract problems and remain open to future improvements or extensions. This principle is closely linked to Generic Code Encapsulates What Changes, because business-specific logic tends to be the most volatile aspect of a project.
Avoid IF Statements in Generic Code
For more details on avoiding if
statements, see Beyond If Statements: Ways to avoid IFs.
I’ve lost track of how many times I’ve heard “this is generic code,” yet found numerous if
statements addressing specific business cases. The moment you include such conditional logic in a “generic” component, it’s no longer truly generic.
Instead of adding if
statements for specific use cases, find an abstraction that addresses the underlying problem – not just your immediate one.
if (accountType == 'Individual') { // ... } else if (accountType == 'Partnership') { // ... } else if (accountType == 'Customer') { // ... }
Use More Generic Naming Conventions
Another frequent mistake is using names for properties, methods, or classes that reference a specific business scenario, or creating a component that could be more general but restricting it to a single use case.
❌ Incorrect
import { LightningElement, api } from 'lwc'; export default class CategoryPills extends LightningElement { @api categories = []; // [{ categoryLabel: '', categoryValue: '' }] }
In this example, the component is called CategoryPills
. The name and property suggest it’s only meant for “categories.” What if you need a similar set of pills for table filters or other use cases?
✅ Correct
import { LightningElement, api } from 'lwc'; export default class Pills extends LightningElement { @api pills = []; // [{ label: '', value: '' }] }
Generic naming allows you to reuse the component for different business scenarios.
<!-- categoryPills.html --> <template> <c-pills pills={categories}></c-pills> </template>
<!-- filters.html --> <template> <c-pills pills={appliedFilters}></c-pills> </template>
Where Should Business Logic Go?
This is where the Open-Closed Principle becomes important: your code should be open for extension but closed for modification. Structure your code so that adding logic for corner cases doesn’t force you to change the original generic code. Developers who need extra functionality can implement it in a separate class.
Apex
Virtual Classes
One of the easiest ways to achieve this in Apex is by using virtual classes. A virtual class can be instantiated for most standard use cases; if a developer requires more functionality, they can extend the virtual class without modifying your original code.
public virtual inherited sharing class SOQL implements Queryable { // ... // SOQL covers only generic cases // The class doesn't contain logic for any specific object // It operates on an abstraction: SObject }
public inherited sharing class SOQL_Account extends SOQL { // ... public SOQL_Account byId(Id accountId) { // ... } public SOQL_Account byParentId(Id parentId) { // ... } public SOQL_Account byIndustry(String industry) { // ... } // ... }
Design Patterns
Design patterns are excellent for separating generic code from concrete implementations. A straightforward example is the Strategy Pattern, which clearly demonstrates how to split out business logic. Below is a generic approach using BonusStrategy and BonusService. Developers who want to extend the code only need to create a new strategy class.
public interface BonusStrategy { Decimal calculateBonus(Decimal baseSalary); } public class ChristmasBonusStrategy implements BonusStrategy { public Decimal calculateBonus(Decimal baseSalary) { return baseSalary * 1.15; } } public class PerformanceBonusStrategy implements BonusStrategy { public Decimal calculateBonus(Decimal baseSalary) { return baseSalary * 1.10; } } public with sharing class BonusService { private BonusStrategy strategy; public BonusService(BonusStrategy strategy) { this.strategy = strategy; } public Decimal applyBonus(Decimal baseSalary) { return strategy.calculateBonus(baseSalary); } } public with sharing class BonusExample { public static void execute() { BonusStrategy christmasStrategy = new ChristmasBonusStrategy(); BonusService christmasService = new BonusService(christmasStrategy); Decimal christmasBonus = christmasService.applyBonus(4000); } }
LWC
Slots
Slots are powerful because they allow you to pass markup from a parent to a child component. They are extremely useful for creating generic code. Here’s a simple example:
Here is our generic code:
<!-- customRelatedList.html --> <template> <div class="slds-card slds-card_boundary"> <div class="slds-card__header slds-grid"> <!-- code here --> <div class="slds-no-flex"> <slot name="actions"></slot> </div> </div> <div class="content"> <slot name="content"></slot> </div> <footer class="footer"> <slot name="footer"></slot> </footer> </div> </template>
Specific Usage:
<template> <c-custom-related-list> <div class="slds-grid" slot="actions"> <lightning-button label="View" onclick={handleView}> </lightning-button> <lightning-button label="Delete" onclick={handleDelete}> </lightning-button> </div> <div slot="contant"> <c-my-accounts-datatable></c-my-accounts-datatable> </div> </c-custom-related-list> </template>
We never modify customRelatedList; we simply pass what we need via slots.
api
Using the @api decorator allows you to expose public properties of an LWC component. This approach lets you create a component that provides configurable properties, while the business logic of using those properties remains elsewhere.
<template> <lightning-datatable key-field="id" data={accounts} columns={accountColumns}> </lightning-datatable> </template>
Generic Code Is Not Overcomplicated
aka Single Responsibility Principle
aka Keep It Simple, Stupid (KISS)
Custom metadata, JSON configurations, design patterns, utilities, services, selectors, unit of work, enterprise patterns – your code seems to have everything one could ever dream of. But is it really worth it?
Here’s the thing:
- When you need dinner, you don’t necessarily need a Thermomix.
- When you need to dig a hole for a plant, you don’t necessarily need a digger.
Many developers believe “generic code” means endless possibilities and configurations. That’s not the case. Start simple, keep it simple, then adjust and evolve over time.
Don’t get me wrong – everything has its place, but sometimes we adopt fancy approaches without critical thinking. We end up with code overloaded by design patterns, metadata options, and configurations, making it cumbersome to use.
Try to solve the problem with the right tool. Don’t over-engineer or overshoot your requirements; let your code evolve as needed.
Generic Code Does Not Mean Metadata-Driven Code
It’s Monday, a new sprint has begun, and you have a new task: create a custom datatable that can be used across every team in the company. You’re excited about making your code “generic,” and the idea of telling your mom “Mom, my code is so generic!” makes you smile.
Your first thought might be:
Generic code = Configurable code = Custom metadata.
How do you store large amounts of data in custom metadata?
- Custom fields for a static structure? Nah, too basic.
- Then the idea strikes: a long text area field with JSON!
You end up with JSON like this:
{ "header": "Team A Datatable", "objectApiName": "Document__c", "columns": [ { "label": "Name", "fieldName": "Name", "type": "text" }, { "label": "Type", "fieldName": "Type", "type": "text" }, // ... ], "globalActions": [ { "type": "button", "label": "Upload", "name": "upload" }, { "type": "search", "label": "Search...", "name": "search" } ], "search": { "fields": [ "Name", "Description" ] }, // ... }
The Problem
Unpredictable, Hard-to-Debug Code
Dynamically injecting custom metadata into a component can make it unpredictable and difficult to debug.
Lack of Standardization
When teams keep adding fields to the JSON, the component becomes harder to maintain. It’s not clear which fields are required or optional, and small changes can break the entire setup.
Unrealistic Expectations of “Configuration”
While the goal is often to let non-developers update these configurations in production, that rarely happens. In practice, most people are reluctant to edit metadata live, because a single mistake can lead to system-breaking behavior. Consequently, the “configurable” metadata is left untouched.
Don’t get me wrong – there are well-designed, metadata-driven solutions out there, but they’re in the minority.
Ask yourself: Will anyone actually change this metadata? If the answer is “probably not,” then perhaps storing the configuration directly in the component is a better approach, allowing for easier debugging and maintenance.
❌ Less Ideal
<!-- genericDatatable.xml --> <?xml version="1.0" encoding="UTF-8"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata"> <apiVersion>63.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__AppPage</target> <target>lightning__RecordPage</target> <target>lightning__HomePage</target> </targets> <targetConfig> <targetConfig> <property name="configName" label="Metadata Name" type="String" /> </targetConfig> </targetConfig> </LightningComponentBundle>
This can lead to highly dynamic logic that’s harder to troubleshoot.
✅ Better
<!-- accountDatatable.html --> <template> <c-generic-datatable columns={columns} data={accounts} ></c-generic-datatable> </template>
<!-- contactsDatatable.html --> <template> <c-generic-datatable columns={columns} data={contacts} ></c-generic-datatable> </template>
"Hardcoding" certain values in code isn’t always bad. It often simplifies debugging and makes the code more understandable. Remember the Cognitive Capacity concept: extremely dynamic code can be harder to follow. “Hardcoding” (or maintaining a static approach) can sometimes enhance readability and reduce complexity.
Generic code doesn’t always mean metadata-driven code. A well-structured, static component can be just as maintainable, if not more so, while still providing enough flexibility for real-world use.
Generic Code Does Not Mean Utils, Services, and Helpers
aka Single Responsibility Principle
You’ve identified repetitive code throughout your project and want to make it “generic” so that other developers can reuse it. That’s a noble goal!
What’s your next step?
Many developers create a Helper.cls
, Service.cls
, or Utils.cls
class to store this “generic” code, believing that the names alone – “Helper,” “Service,” or “Utils” – will guarantee reusability. But is that truly the case?
Classes named “Helper,” “Service,” or “Utils” often become a dumping ground for messy, repetitive code. Developers keep adding methods, causing these classes to grow unwieldy, hard to read, and prone to merge conflicts:
public with sharing class Utils { public static Boolean isNullOrEmpty(String input) { return input == null || input.trim().length() == 0; } public static Boolean isNullOrBlank(String input) { return input == null || input.trim().length() == 0; } public static String safeSubstring(String input, Integer start, Integer length) { if (input == null) return ''; Integer endIndex = Math.min(start + length, input.length()); if (start < 0 || start > input.length() || endIndex < 0) { return ''; } return input.substring(start, endIndex); } public static Date parseDateSafe(String dateStr) { if (isNullOrEmpty(dateStr)) { return null; } try { return Date.valueOf(dateStr); } catch (Exception e) { // If dateStr isn't in YYYY-MM-DD format, parsing fails return null; } } public static String toJson(Object obj) { return JSON.serialize(obj); } public static Object fromJson(String jsonString, Type apexType) { if (isNullOrEmpty(jsonString)) { return null; } return JSON.deserialize(jsonString, apexType); } public static Map<Id, SObject> toIdMap(List<SObject> records) { Map<Id, SObject> results = new Map<Id, SObject>(); if (records == null) return results; for (SObject rec : records) { if (rec.Id != null) { results.put(rec.Id, rec); } } return results; } public static Set<Id> toIdSet(List<SObject> records) { Set<Id> ids = new Set<Id>(); if (records == null) return ids; for (SObject rec : records) { if (rec.Id != null) { ids.add(rec.Id); } } return ids; } public static List<Id> removeNullIds(List<Id> idList) { List<Id> cleaned = new List<Id>(); if (idList != null) { for (Id oneId : idList) { if (oneId != null) { cleaned.add(oneId); } } } return cleaned; } public static void debug(String logLabel, Object info) { System.debug(logLabel + ': ' + String.valueOf(info)); } public static String removeSpecialChars(String input) { if (isNullOrEmpty(input)) return ''; return input.replaceAll('[^a-zA-Z0-9\\s\\-\\_]+', ''); } }
Split Logic Based on Functionality
The Single Responsibility Principle states that developers should group components that change for the same reason. Don’t be afraid to split logic by topic or functionality. Create classes dedicated to one specific task, with logic that focuses on one purpose.
public with sharing class RecordTypesInfo { // ... public static Id getId(SObjectType objectType, String recordTypeDeveloperName) { // ... } public static String getDeveloperName(SObjectType objectType, Id recordTypeId) // ... } public static Schema.RecordTypeInfo byDeveloperName(SObjectType objectType, String recordTypeDeveloperName) { // ... } }
public with sharing class ProfileInfo { private final static Map<String, Profile> NAME_TO_PROFILE = new Map<String, Profile>(); public static Id toId(String profileName) { return getByName(profileName).Id; } public static Profile getByName(String profileName) { Profile profile = NAME_TO_PROFILE.get(profileName); if (profile == null) { profile = [ SELECT Id, Name FROM Profile WHERE Name = :profileName LIMIT 1 ]; NAME_TO_PROFILE.put(profileName, profile); } return profile; } }
public with sharing CurrentUserInfo { private static User cachedUserInfo { get { if (cachedUserInfo == null) { cachedUserInfo = [ SELECT Id, .... FROM User WHERE Id = :UserInfo.getUserId() ]; } return cachedUserInfo; } private set; } public static Id getContactId() { return cachedUserInfo?.ContactId; } public static Account getPrimaryAccount() { return cachedUserInfo?.Contact?.Account; } }
Constants
Another example is handling constants. A common approach is to create a single “Constants” class, like:
❌ Centralizing All Constants
public class Constants { public static final String ACCOUNT_PROSPECT_TYPE = 'Prospect'; public static final String ACCOUNT_CUSTOMER_DIRECT_TYPE = 'Customer - Channel'; public static final String CONTACT_WEB_LEAD_SOURCE = 'Web'; public static final String CONTACT_PHONE_INQUIRY_LEAD_SOURCE = 'Phone Inquiry'; public static final String SYSTEM_ADMINISTRATOR_PROFILE = 'System Administrator'; public static final String CUSTOMER_COMMUNITY_LOGIN_USER_PROFILE = 'Customer Community Login User'; // ... }
✅ Grouping Constants by Functionality
Here, constants are grouped in different classes, making the code easier to read and easier to locate. The final usage – Consts.ACCOUNT.TYPE.PROSPECT
– also clearly identifies the constant’s purpose.
// ContactConsts.cls public class ContactConsts { public static final ContactConsts INSTANCE = new ContactConsts(); // signleton public final String API_NAME = Contact.sObjectType.getDescribe().getName(); public final LeadSource LEAD_SOURCE = new LeadSource(); private class LeadSource { public final String OTHER = 'Other'; public final String PARTNER_REFERRAL = 'Partner Referral'; public final String PHONE_INQUIRY = 'Phone Inquiry'; public final String PURCHASED_LIST = 'Purchased List'; public final String WEB = 'Web'; } } // ProfileConsts.cls // AccountConsts.cls // OportunityConsts.cls
// Consts.cls public with sharing class Consts { public static final ContactConsts CONTACT { get { return ContactConsts.INSTANCE; } } public static final ProfileConsts PROFILE { get { return ProfileConsts.INSTANCE; } } // ... }
System.debug(Consts.ACCOUNT.TYPE.PROSPECT); // 'Prospect' System.debug(Consts.ACCOUNT.RATING.HOT); // 'Hot' System.debug(Consts.CONTACT.API_NAME); // 'Contact' System.debug(Consts.CONTACT.LEAD_SOURCE.WEB); // 'Web' System.debug(Consts.OPPORTUNITY.TYPE.EXISTING_CUSTOMER_DOWNGRADE); // 'Existing Customer - Downgrade'
For more on Constants in Apex, see: Constants in Apex.
Generic Code Does Not Cover Every Possible Case
aka Pareto Rule (80/20)
aka Single Responsibility Principle
aka Open-Closed principle
aka Hick’s Law
The Pareto rule (80/20) suggests that roughly 80% of outcomes come from 20% of causes. You can apply this same principle to your generic code. Sometimes, you only need 20% of your code to handle 80% of the scenarios. Meanwhile, adding the other 80% of code just to address the remaining 20% of use cases may not always be worthwhile. You still need to maintain it and fix all bugs.
Focus on what matters – the primary 80% of your use cases. Make that part of your code clean, predictable, and intuitive.
Remember: The best code is the code that never needs to be written.
But what about the remaining 20%?
Make Your Code Easy to Extend
Apply the Open-Closed Principle. Keep your code open for extension.
Generic code does not need to offer endless possibilities. Human cognitive capacity is limited. Instead of continually adding more options to your “generic” code to cover every edge case, allow developers to extend your logic. Focus on a clean, intuitive base for the critical 80% of scenarios.
A great approach might be the Decorator pattern, which lets you extend a class’s behavior without modifying the original code.
Default Configurations (Revisited)
A default configuration can handle 80% of cases, while allowing overrides for the remaining 20%. Suppose you have a custom data table that includes several predefined actions (view, edit, delete, download). Another team might need a very specific action – like integrating with a third-party system.
You could add the entire integration to your generic code, but that would double its complexity to handle a single edge case. Don’t modify your “generic” code (open-closed principle). Instead, create a way to inject custom actions. The data table’s default actions cover 80% of scenarios, while the remaining 20% can be achieved through configuration and overrides.
Think Out Of The Box
A problem well-stated is a problem half-solved.
Many issues can disappear if you approach them from a different angle. No problem means no code is needed to solve it.
Often, we pile every requirement into our “generic” code without considering how frequently the use case arises. Maybe that edge case should be covered by new code that extends existing functionality, rather than by complicating your generic solution.
Example: Suppose you have an Apex Query Builder class that constructs SOQL queries. The business requests a feature to export the resulting data to .csv. Should you add CSV export functionality to the Query Builder class used by multiple teams? Probably not – CSV export is a niche requirement. Instead, create a separate module that accepts a Query Builder instance, generates a .csv
, and handles export. This keeps your generic code focused and uncluttered.
How to Write Generic Code?
1. Define the Abstract Problem
I love this quote, so let’s repeat it:
“A problem well-stated is a problem half-solved.”
Ask yourself: What abstract problem are you trying to solve? Begin by examining your concrete task, then progressively zoom out to identify the broader challenge. Move from specific details to general concepts.
2. Start from the End
This is the most important phase.
Create a rough outline of how your code will be used. Define the method names that developers will call:
- Is the naming intuitive?
- Does it read like plain English?
- Would someone new to the project immediately understand it?
- How many steps my code requires to start using it?
For example, while working on the Cache Manager, I initially wrote:
CacheManager.getOrgCache('CacheName').put('SomeKey', 'SomeValue');
Then I realized that other developers might not know the cache name. They’d have to ask around, check setup, or dig into existing code – too many steps. Since there aren’t many cache instances in the org, I decided to keep that logic inside the CacheManager class:
public with sharing class CacheManager { // ... public final static Cacheable SOQLOrgCache { get { return getOrgCache('SOQL'); } } public final static Cacheable SOQLSessionCache { get { return getSessionCache('SOQL'); } } // ... }
Now using it is incredibly simple:
CacheManager.SOQLOrgCache.put('SomeKey', 'SomeValue');
Developers don’t need to worry about the cache name at all.
3. Consider Edge Cases and Bottlenecks
Determine which aspects of your code are static – those that remain consistent and repeat – and which aspects vary or are unusual.
Example: Creating a custom datatable.
Static elements:
- Specifying columns
- Providing data
- Defining global actions
- Pagination
Elements that varies:
- The data source (Salesforce, AWS, etc.)
Once you identify these, you can build a reusable core for the static parts and delegate record retrieval (for example, from external systems) to a separate module or to any developer who wants to integrate your code.
Final Thoughts
Generic code isn’t just about flexibility – it’s about balancing simplicity and robustness so that multiple teams and use cases can benefit.
What does generic code do?
- Identifies the Abstract Problem
- Presents a Clear Interface
- Encapsulates Implementation Details
- Remains Easy to Adopt
- Avoids Over-Engineering
Ultimately, generic code lets you solve a problem once and then reapply it consistently across different contexts. It reduces duplication, enforces consistency, and enables your team to collaborate effectively – without creating a maintenance nightmare.
Note: This post was not written by AI. I only used AI to help refine my grammar because I am not a native speaker.